Integrate CCAvenue Payment Gateway with Laravel
Laravel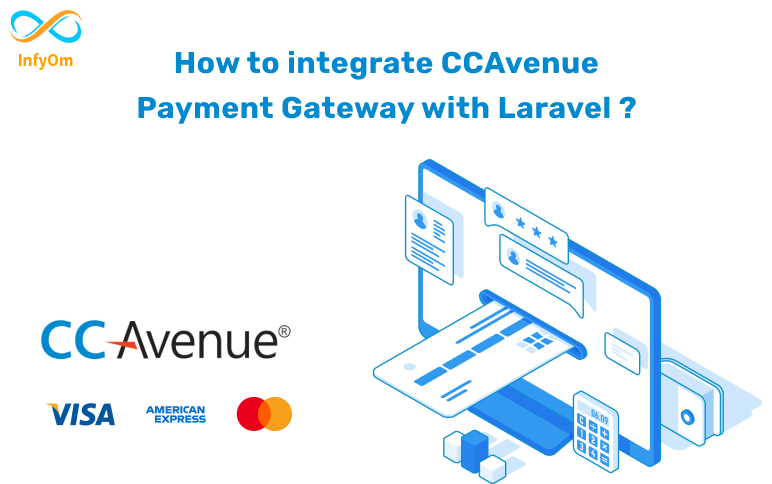
This is the hardest thing as a developer I found, as there is no official documentation available to integrate the CCAvenue. Also, you will not find any tutorial or blog from which you can do the implementation.
So, in this tutorial, we are going to cover how we can integrate the CCAvenue payment gateway with Laravel.
web.php
Route::post('purchase', [CCAvenueController::class, purchaseSubscription']);
CCAvenueController.php
public function purchaseSubscription(Request $request)
{
$input = $request->all();
$input['amount'] = $data['grandTotal'];
$input['order_id'] = "123XSDDD456";
$input['currency'] = "INR";
$input['redirect_url'] = route('cc-response');
$input['cancel_url'] = route('cc-response');
$input['language'] = "EN";
$input['merchant_id'] = "your-merchant-id";
$merchant_data = "";
$working_key = config('cc-avenue.working_key'); //Shared by CCAVENUES
$access_code = config('cc-avenue.access_code'); //Shared by CCAVENUES
$input['merchant_param1'] = "some-custom-inputs"; // optional parameter
$input['merchant_param2'] = "some-custom-inputs"; // optional parameter
$input['merchant_param3'] = "some-custom-inputs"; // optional parameter
$input['merchant_param4'] = "some-custom-inputs"; // optional parameter
$input['merchant_param5'] = "some-custom-inputs"; // optional parameter
foreach ($input as $key => $value) {
$merchant_data .= $key . '=' . $value . '&';
}
$encrypted_data = $this->encryptCC($merchant_data, $working_key);
$url = config('cc-avenue.url') . '/transaction/transaction.do?command=initiateTransaction&encRequest=' . $encrypted_data . '&access_code=' . $access_code;
return redirect($url);
}
Manage Callback
public function ccResponse(Request $request)
{
try {
$workingKey = config('cc-avenue.working_key'); //Working Key should be provided here.
$encResponse = $_POST["encResp"];
$rcvdString = $this->decryptCC($encResponse, $workingKey); //Crypto Decryption used as per the specified working key.
$order_status = "";
$decryptValues = explode('&', $rcvdString);
$dataSize = sizeof($decryptValues);
}
Other Encryption functions
public function encryptCC($plainText, $key)
{
$key = $this->hextobin(md5($key));
$initVector = pack("C*", 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09, 0x0a, 0x0b, 0x0c, 0x0d, 0x0e, 0x0f);
$openMode = openssl_encrypt($plainText, 'AES-128-CBC', $key, OPENSSL_RAW_DATA, $initVector);
$encryptedText = bin2hex($openMode);
return $encryptedText;
}
public function decryptCC($encryptedText, $key)
{
$key = $this->hextobin(md5($key));
$initVector = pack("C*", 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09, 0x0a, 0x0b, 0x0c, 0x0d, 0x0e, 0x0f);
$encryptedText = $this->hextobin($encryptedText);
$decryptedText = openssl_decrypt($encryptedText, 'AES-128-CBC', $key, OPENSSL_RAW_DATA, $initVector);
return $decryptedText;
}
public function pkcs5_padCC($plainText, $blockSize)
{
$pad = $blockSize - (strlen($plainText) % $blockSize);
return $plainText . str_repeat(chr($pad), $pad);
}
public function hextobin($hexString)
{
$length = strlen($hexString);
$binString = "";
$count = 0;
while ($count < $length) {
$subString = substr($hexString, $count, 2);
$packedString = pack("H*", $subString);
if ($count == 0) {
$binString = $packedString;
} else {
$binString .= $packedString;
}
$count += 2;
}
return $binString;
}
That's it.
So that is how you can integrate the CCAvenue Payment Gateway with Laravel.